This sample android program shows you how write and read a file from SD Card
in Android. In this program four buttons are shown and a Edit box. When you type
some text into the edit box and click, Save to SD Card button, the text is saved
to a text file and saved to the SD Card. When you click clear button, the edit
box contents are cleared. When you click, Read Sd card button the file is read
from the SD card and the contents are copied to the edit box.
The FileDemo2.java file is as follows:
The main.xml file in your res/layout folder is as follows:
The FileDemo2.java file is as follows:
package com.javasamples;
import java.io.*;
import android.app.Activity;
import android.os.Bundle;
import android.view.*;
import android.view.View.OnClickListener;
import android.widget.*;
public class FileDemo2 extends Activity {
// GUI controls
EditText txtData;
Button btnWriteSDFile;
Button btnReadSDFile;
Button btnClearScreen;
Button btnClose;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
// bind GUI elements with local controls
txtData = (EditText) findViewById(R.id.txtData);
txtData.setHint("Enter some lines of data here...");
btnWriteSDFile = (Button) findViewById(R.id.btnWriteSDFile);
btnWriteSDFile.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
// write on SD card file data in the text box
try {
File myFile = new File("/sdcard/mysdfile.txt");
myFile.createNewFile();
FileOutputStream fOut = new FileOutputStream(myFile);
OutputStreamWriter myOutWriter =
new OutputStreamWriter(fOut);
myOutWriter.append(txtData.getText());
myOutWriter.close();
fOut.close();
Toast.makeText(getBaseContext(),
"Done writing SD 'mysdfile.txt'",
Toast.LENGTH_SHORT).show();
} catch (Exception e) {
Toast.makeText(getBaseContext(), e.getMessage(),
Toast.LENGTH_SHORT).show();
}
}// onClick
}); // btnWriteSDFile
btnReadSDFile = (Button) findViewById(R.id.btnReadSDFile);
btnReadSDFile.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
// write on SD card file data in the text box
try {
File myFile = new File("/sdcard/mysdfile.txt");
FileInputStream fIn = new FileInputStream(myFile);
BufferedReader myReader = new BufferedReader(
new InputStreamReader(fIn));
String aDataRow = "";
String aBuffer = "";
while ((aDataRow = myReader.readLine()) != null) {
aBuffer += aDataRow + "\n";
}
txtData.setText(aBuffer);
myReader.close();
Toast.makeText(getBaseContext(),
"Done reading SD 'mysdfile.txt'",
Toast.LENGTH_SHORT).show();
} catch (Exception e) {
Toast.makeText(getBaseContext(), e.getMessage(),
Toast.LENGTH_SHORT).show();
}
}// onClick
}); // btnReadSDFile
btnClearScreen = (Button) findViewById(R.id.btnClearScreen);
btnClearScreen.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
// clear text box
txtData.setText("");
}
}); // btnClearScreen
btnClose = (Button) findViewById(R.id.btnClose);
btnClose.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
// clear text box
finish();
}
}); // btnClose
}// onCreate
}// AndSDcard
The output of this program will be as shown in the android emulator below.
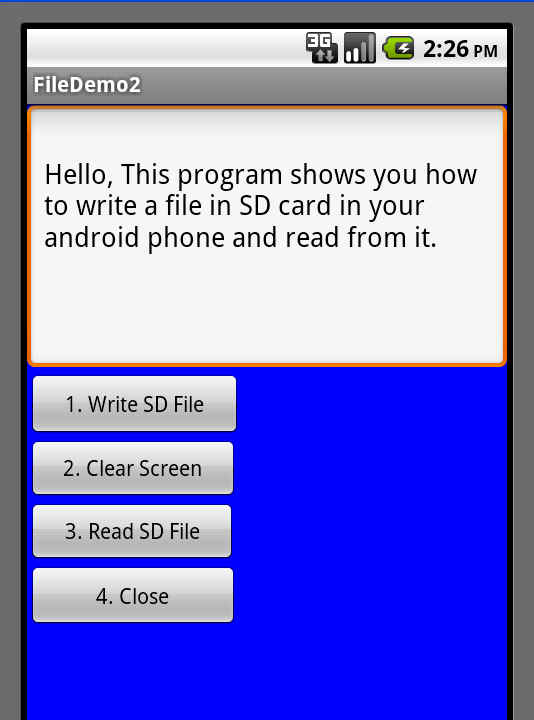
The main.xml file in your res/layout folder is as follows:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
android:id="@+id/widget28"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#ff0000ff"
android:orientation="vertical"
xmlns:android="http://schemas.android.com/apk/res/android"
>
<EditText
android:id="@+id/txtData"
android:layout_width="fill_parent"
android:layout_height="180px"
android:textSize="18sp" />
<Button
android:id="@+id/btnWriteSDFile"
android:layout_width="143px"
android:layout_height="44px"
android:text="1. Write SD File" />
<Button
android:id="@+id/btnClearScreen"
android:layout_width="141px"
android:layout_height="42px"
android:text="2. Clear Screen" />
<Button
android:id="@+id/btnReadSDFile"
android:layout_width="140px"
android:layout_height="42px"
android:text="3. Read SD File" />
<Button
android:id="@+id/btnClose"
android:layout_width="141px"
android:layout_height="43px"
android:text="4. Close" />
</LinearLayout>
More details
Brilliant work.good choice..Keep it up.I like your blog talent.Supper.
ReplyDeleteThis is one of the important and good post.I like your blog details.Thanks for your support.Good.
ReplyDelete