GCM is a (free) service that allows developers to push 2 types of messages from their application servers to any number of Android devices registered with the service:
"Collapsible" means that the most recent message overwrites the previous one. A "send-to-sync" message is used to notify a mobile application to sync its data with the server. In case the device comes online after being offline for a while, the client will only get the most recent server message.
- Collapsible, "send-to-sync" messages
- Non-collapsible messages with a payload up to 4K in size
If you want to add push notifications to your Android applications, the Getting Started guide will walk you through the set up process step by step, even supplying you with a two-part Demo Application (client + server) that you can just install and play around with. The set-up process will provide you with the two most essential pieces of information needed to run GCM:
All summarized in the following screen you get after using the Google API console:
- An API key, needed by your server to send GCM push notifications
- A Sender ID, needed by your clients to receive GCM messages from the server
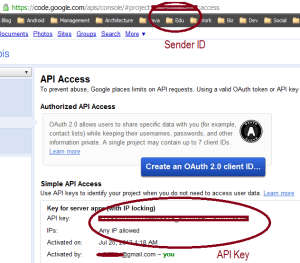
To write both server and client code, the quickest way is to install the Sample Demo Application and tweak it to your needs. In particular, you might want to at least do any of the following:
- Change the Demo's in-memory datastore into a real persistent one
- Change the type and/or the content of push messages
- Change the client automatic device registration on start-up, to a user preference, so that the handset user may have the option to register/un-register for the push notifications.
In your Android project-resources ( res/xml) directory, create a preference.xml file such as this one:
<?
xml
version
=
"1.0"
encoding
=
"utf-8"
?>
<
PreferenceScreen
xmlns:android
=
"http://schemas.android.com/apk/res/android"
>
<
PreferenceCategory
android:title
=
"Push Information"
>
<
EditTextPreference
android:key
=
"sname"
android:summary
=
"Enter the Server Name"
android:title
=
"Name"
/>
<
EditTextPreference
android:key
=
"sip"
android:summary
=
"Enter the Server IP Address"
android:title
=
"IP Address"
/>
<
EditTextPreference
android:key
=
"sport"
android:summary
=
"Enter the Server Port"
android:title
=
"Port"
/>
<
EditTextPreference
android:key
=
"sid"
android:summary
=
"Enter the Sender ID"
android:title
=
"Sender ID"
/>
</
PreferenceCategory
>
<
PreferenceCategory
android:title
=
"Push Settings"
>
<
CheckBoxPreference
android:key
=
"enable"
android:summary
=
"On/Off"
android:title
=
"Enable Server Push"
/>
</
PreferenceCategory
>
</
PreferenceScreen
>
// package here
import
android.os.Bundle;
import
android.preference.PreferenceActivity;
public
class
PushPrefsActivity
extends
PreferenceActivity {
@Override
protected
void
onCreate(Bundle savedInstanceState) {
super
.onCreate(savedInstanceState);
addPreferencesFromResource(R.xml.preferences);
}
}
The above will provide the following UI:
![]() |
![]() |
The "Enable Server Push" checkbox is where your Android application user decides to register for your push messages. Then, it's only a matter of using that preferences class in your main activity, and doing the required input processing. The following skeleton class only shows your own code add-ons to the pre-existing sample application:
The above is pretty much self-explanatory. Now GCM push notifications have been integrated into your existing application. If you are registered, you get a system notification message at each server push, even when your application is not running. Opening up the message will automatically open your application:/ package here import com.google.android.gcm.GCMRegistrar; // other imports here public class MainActivity extends Activity { /** These two should be static imports from a Utilities class*/ public static String SERVER_URL; public static String SENDER_ID; private boolean PUSH_ENABLED; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // other code here... processPush(); } /** Check push on Back button * if PushPrefsActivity is next activity on stack */ @Override public void onResume(){ super.onResume(); processPush(); } /** * Enable user to register/unregister for push notifications * 1. Register user if all fields in prefs are filled AND flag is set * 2. Un-register if flag is un-set and user is registered * */ private void processPush(){ if( checkPushPrefs() && PUSH_ENABLED ){ // register for GCM using the Sample app code } if(! PUSH_ENABLED && GCMRegistrar.isRegisteredOnServer(this) ){ GCMRegistrar.unregister(this); } } /** Check Server Push preferences */ private boolean checkPushPrefs(){ SharedPreferences prefs = PreferenceManager .getDefaultSharedPreferences(this); String name = prefs.getString("sname", ""); String ip = prefs.getString("sip", ""); String port = prefs.getString("sport", ""); String senderID = prefs.getString("sid", ""); PUSH_ENABLED = prefs.getBoolean("enable", false); boolean allFilled = checkAllFilled(name, ip, port, senderID); if( allFilled ){ SENDER_ID = senderID; SERVER_URL = "http://" + ip + ":" + port + "/" + name; } return allFilled; } /** Checks if any number of string fields are filled */ private boolean checkAllFilled(String... fields){ for (String field:fields){ if(field == null || field.length() == 0){ return false; } } return true; } }

GCM is pretty easy to set up, since most of the plumbing work is done for you. A side note: If you like to isolate the push functionality in its own sub-package, be aware that the GCM Service GCMIntentService, provided by the Sample application and responsible for handling GCM messages, needs to be in your main package (as indicated in the-set up documentation)—otherwise GCM won't work.
When communicating with the Sample Server via HTTP Post, the Sample Client does a number of automatic retries using exponential back-off, meaning that the wait period before a retry in case of failure is each time twice the amount of the preceding wait period, up to the maximum number of retries (5 at the time of this writing). You might want to change that if it doesn't suit you. It may not matter that much, though, since those retries are done in a separate thread (using AsyncTask) from the main UI thread, which therefore minimizes the effects on your mobile application's preexisting flow of operations.
From: Tony's Blog.
0 comments
Thanks for your comment